Manual
Table Of Contents
- Table of Contents
- Preface
- 1 Introduction
- 2 Creating and Redistributing LNS Device Plug ins
- 3 How Plug ins Work with Directors
- How Plug ins Are Represented in the LNS Object Server
- How Plug ins are Installed and Made Visible to LNS
- How Plug ins Implement the Registration Command
- How Plug ins Respond to Commands from a Director Other than Registration
- How Directors Launch and Manipulate Plug ins
- What Plug ins Do When They Run in Standalone Mode
- Responding to Property Reads and Writes
- Uninstallation Issues
- Appendix A Standard Plug in Commands
- Appendix B Standard Plug in Properties
- Appendix C Standard Plug in Object Classes
- Appendix D Standard Plug in Exceptions
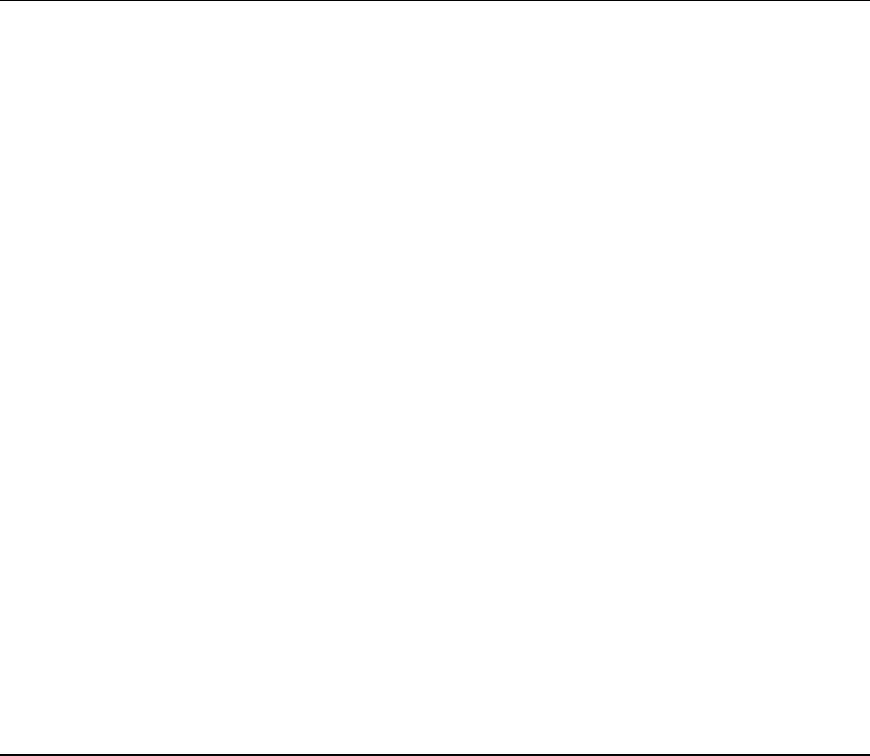
Implementing the Plug-in Server Class
To implement your plug-in server class, modify the source as follows:
1. Add using System.Runtime.InteropServices and using Echelon.LNS.Plugin directives.
2. Add a ComVisible(false) attribute to the class. You will not need to create this class directly from
COM clients.
3. Derive your plug-in server class from the PluginServerBase base class (located in the
Echelon.LNS.Plugin namespace).
4. Add a static Main implementation with an STAThread attribute to the class. Main should create
an instance of your plug-in server class, and then call the base class StartPluginServer method on
this instance, passing in the command-line arguments that were passed into Main and an array of
Type objects, one for each plug-in object class that you will create (typically just the one).
The following code demonstrates how your plug-in server class should appear (note that MyPlugin
represents the name of your plug-in):
using System;
using System.Runtime.InteropServices;
using Echelon.LNS.Plugin;
namespace YourCompany.DevicePlugin
{
[ComVisible(false)]
class MyPluginServer : PluginServerBase
{
[STAThread]
public static void Main(string[] args)
{
Type[] plugIns = { typeof(MyPluginObject) };
MyPluginServer server = new MyPluginServer();
server.StartPluginServer(args, plugIns);
}
}
}
Implementing the Plug-in Object Class
To implement your plug-in object class, modify the source as follows:
1. Add using System.Runtime.InteropServices, using Echelon.LNS.Plugin, and using
Echelon.LNS.Interop directives.
2. Derive your plug-in object class from PluginObjectBase (located in the Echelon.LNS.Plugin
namespace).
3. Add a ComVisible(true) attribute and a public keyword to your plug-in object class to expose it
to COM.
4. Add the additional attributes shown in the following table to your plug-in object class.
Attribute Description Value
Guid Uniquely identifies the plug-in
object to COM.
Generate a unique GUID using the
Create GUID function from the Tools
menu.
ProgId Human readable version of
plug-in identity.
YourCompany.YourProject.MyPlugin,
for example
ClassInterface Disables generation of a default
class interface.
ClassInterfaceType.None